Building efficient user interfaces with React
From the foundations of components, props, and state, to the advanced techniques of rendering optimization and UI design best practices, you'll gain the tools and knowledge to weave remarkable interfaces.
12 June, 2023As technology advances, user interfaces and other app features play a pivotal role in defining the success of digital products, making it crucial to prioritize their development and optimization. Now that we know how to build Progressive Web Applications with React, what about user interfaces in general?
The demand for exceptional frontend development services is on the rise as businesses strive to captivate users with intuitive and visually appealing interfaces, and one of the best ways to do that today is by using React.
So, let’s embark on a quest to unravel the secrets of React's magic. From the foundations of components, props, and state, to the advanced techniques of rendering optimization and UI design best practices, you'll gain the tools and knowledge to weave remarkable interfaces.
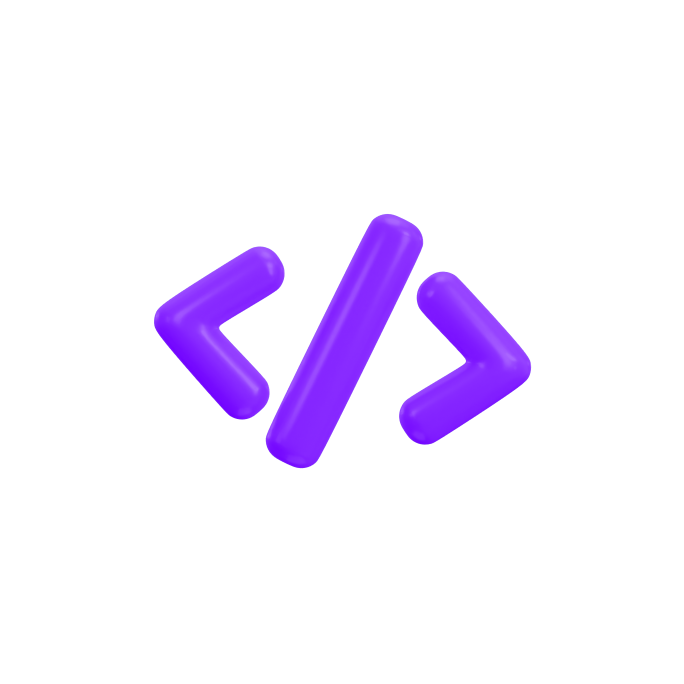
Get developers that understand your business goals
On-time project delivery. Latest coding standards. Data security.
Learn moreBasics of interfaces in React
React revolves around components - self-contained, reusable pieces of code that encapsulate a specific functionality or UI element. Managing and maintaining complex applications is much more efficient by breaking down the user interface into smaller, modular pieces.
In addition to components, React employs a virtual DOM (Document Object Model) for efficient UI rendering. It’s a lightweight representation of the actual DOM, allowing React to efficiently update only the necessary parts of the UI when the underlying data changes, minimizing unnecessary re-renders and improving performance.
React also follows a declarative programming paradigm, where developers describe how the UI should look based on the current state of the application. It then handles the updates and ensures the UI remains in sync with the data.
Furthermore, React provides a unidirectional data flow, meaning that data flows in a single direction within the component hierarchy. This simplifies data management and reduces the likelihood of bugs caused by conflicting data states.
Components, props, and state
Components, props, and state form the bedrock of React's architecture, enabling the creation of dynamic and interactive user interfaces. They can be simple, like a button, or complex, like a form. Components act as building blocks and are composed of two essential elements: props and state.
Props, short for properties, allow data to be passed from a parent component to its child components, enabling the dynamic customization of components based on varying data inputs. Props are read-only and are passed down the component tree, influencing the behavior and appearance of components at different levels.
On the other hand, state represents the internal state of a component and can be updated throughout its lifecycle. It allows components to handle and respond to user interactions, manage data changes, and trigger updates in the UI. State is managed within a component and can be modified using the setState() method, triggering re-rendering and reflecting the updated state.
Building user interfaces
Here are the fundamental principles and techniques that enable the creation of captivating and functional designs with React.
Using React components
There are two primary types of components: functional and class.
Functional components are JS functions that return JSX (JavaScript XML) elements, describing the structure and content of the UI. They're primarily used for rendering static UI based on props and are suitable for most scenarios. They are also called "stateless" because they don't manage state.
Here's an example of a functional component:
import React from 'react';
function MyComponent(props) {
return <div>Hello, {props.name}!</div>;
}
On the other hand, class components are JavaScript classes that extend React's Component class. They introduce the concept of state and lifecycle methods, allowing you to manage dynamic UI elements and handle component lifecycle events.
Here's an example of a class component:
import React from 'react';
class MyComponent extends React.Component {
render() {
return <div>Hello, {this.props.name}!</div>;
}
}
By utilizing these component types, we can modularize our UI, breaking it down into smaller, self-contained pieces. This modular approach enhances code reusability and maintainability, as components can be easily composed and reused across different parts of the application.
Speaking of the previously mentioned JSX. It’s one of the defining features of React - a syntax extension that allows us to write HTML-like code within JavaScript. JSX makes it easier to visualize and construct UI components by providing a familiar syntax. Here's an example of JSX:
const element = <div className="container">Hello, World!</div>;
The <div> element is written in JSX syntax and can contain attributes like className, which corresponds to the class attribute in HTML.
React components also have lifecycle methods that you can override to perform actions at specific stages of a component's life. For instance, componentDidMount is called when a component is rendered for the first time, providing an opportunity to fetch data or set up event listeners.
Similarly, componentDidUpdate is invoked when the component updates, enabling us to handle state changes or perform side effects. Finally, componentWillUnmount allows us to clean up resources and perform necessary actions before a component is unmounted from the DOM.
Styling efficient React components
CSS-in-JS libraries, such as styled-components and CSS modules, provide ways to write and organize CSS within JavaScript or JSX code. They generate unique class names or inline styles for each component, ensuring that styles are isolated and don't clash with other components.
Other advantages include improved code maintainability and reusability. In the first case, by colocating CSS styles with their corresponding components, it becomes easier to understand and maintain the codebase. Reusability means they often provide mechanisms for style reuse, such as defining and extending shared style components or utilizing theme providers.
Next, let's talk about CSS preprocessors and the efficient organization of styles. CSS preprocessors like Sass (Syntactically Awesome StyleSheets) or Less (Leaner CSS) extend the capabilities of traditional CSS and provide a more organized and reusable approach to styling:
- They allow the use of variables, which can store commonly used values such as colors, font sizes, or spacing, enabling consistent styling and making it easy to update styles globally by modifying variable values.
- They support nesting of CSS rules, allowing you to write more concise and readable code. Nested selectors reduce repetition and make the relationships between elements clearer.
- They include mixins, the reusable blocks of CSS properties and values. They enable you to define and reuse styles across multiple selectors, reducing duplication and promoting code modularity.
- Preprocessors also introduce functions and operators that facilitate complex calculations, color manipulation, and other operations, enhancing the flexibility and expressiveness of your stylesheets.
- Finally, they enable importing and organizing stylesheets into separate files, making it easier to manage and modularize styles across different components or pages.
And last but not least, responsive design. It ensures your UI adapts and looks great across various screen sizes and devices. Here are the key techniques:
- Media queries. They allow you to apply different styles based on the characteristics of the device or viewport. By defining breakpoints (specific screen sizes), you can change the layout, font sizes, spacing, or even hide/show elements to optimize the user experience.
- Fluid layouts. Instead of using fixed pixel values for widths and heights, employing relative units like percentages or viewport-based units (e.g., vw, vh) enables fluid layouts that adjust dynamically to different screen sizes. This helps maintain proportionate and flexible designs.
- Mobile-first approach. Building your UI starting from a mobile perspective and then progressively enhancing it for larger screens is a recommended approach. Your design will be optimized for smaller screens and avoid unnecessary bloat on mobile devices.
- Responsive images. Use CSS techniques (e.g., max-width: 100%) or HTML attributes (e.g., srcset and sizes) to optimize image loading and ensure appropriate image sizes are displayed based on device capabilities and screen sizes.
- Flexbox and CSS Grid. These CSS models enable you to build flexible and responsive grids, handle alignment and positioning, and control the flow of elements across different screen sizes.
Making components that are reusable
Building reusable components in React is essential for creating maintainable and scalable applications. Here are key principles and techniques for achieving component reusability:
- Each component should have a clear purpose for easier understanding, testing, and reusability.
- Use props to configure and customize components based on different requirements.
- Identify common logic and extract it into separate functions or custom hooks to promote code reuse and reduce duplication.
- Share data across components without explicit prop passing using React Context, simplifying access to shared information.
- Utilize higher-order components (HOCs) to wrap components with additional functionality, and use render props to enable component customization and data sharing through functions passed as props.
One more technique for simplifying component hierarchy and improving code readability has to do with prop drilling and lifting state.
Prop drilling occurs when props need to be passed through multiple intermediate components to reach a deeply nested component that requires them, leading to a cluttered codebase.
Lifting state moves the shared state up to a common ancestor component, making it accessible to the necessary components without excessive prop drilling.
Optimizing rendering performance
Creating fast and responsive React applications also calls for optimizing rendering performance. Let's go through the fundamental concepts of this process.
When a component's state or props change, React creates a new virtual DOM tree. It then performs a diffing process to identify the minimal set of changes needed to update the actual DOM efficiently. By working with the Virtual DOM instead of directly manipulating the real DOM, React minimizes the number of actual DOM updates, resulting in improved performance.
Then, React's reconciliation algorithm determines the differences between the previous and new virtual DOM trees. It identifies added, removed, or modified components and their corresponding DOM elements. React optimizes this process by applying the changes in batches rather than updating the DOM after each individual change.
Finally, use memoization techniques like shouldComponentUpdate or React.memo to prevent unnecessary re-renders. Consider also using React.PureComponent or React.memo to automatically handle prop comparisons and avoid unnecessary updates. These techniques reduce rendering overhead and improve the overall performance of your React application.
Best practices for React UI design
Here are the four proven best practices for designing user interfaces with React.

Keeping the UI simple
Simplifying the user interface ensures users can effortlessly navigate and understand your application. By eliminating unnecessary clutter, focusing on essential elements, and presenting information in a clear and organized manner, you create an intuitive and user-friendly interface.
Build an interactive user experience
An interactive user experience captivates and engages users, creating a memorable and enjoyable interaction. Incorporate interactive elements such as animations, transitions, and intuitive gestures to provide feedback and guide users through the application. Foster a sense of exploration and responsiveness and, in turn, enhance user satisfaction and make the UI more compelling.
Make use of state
Leveraging state management in React allows you to create dynamic and responsive UIs. By utilizing state to store and update data, you can build components that adapt to user input and reflect changes. Whether it's form validation, dynamic content rendering, or handling user interactions, effectively managing state enhances the interactivity and functionality of your UI.
Use modern libraries and frameworks
Leverage existing tools and solutions that enhance development efficiency and UI capabilities. React's vast ecosystem provides access to libraries and frameworks like Redux, React Router, and Material-UI, which offer ready-made solutions for state management, routing, and UI components.
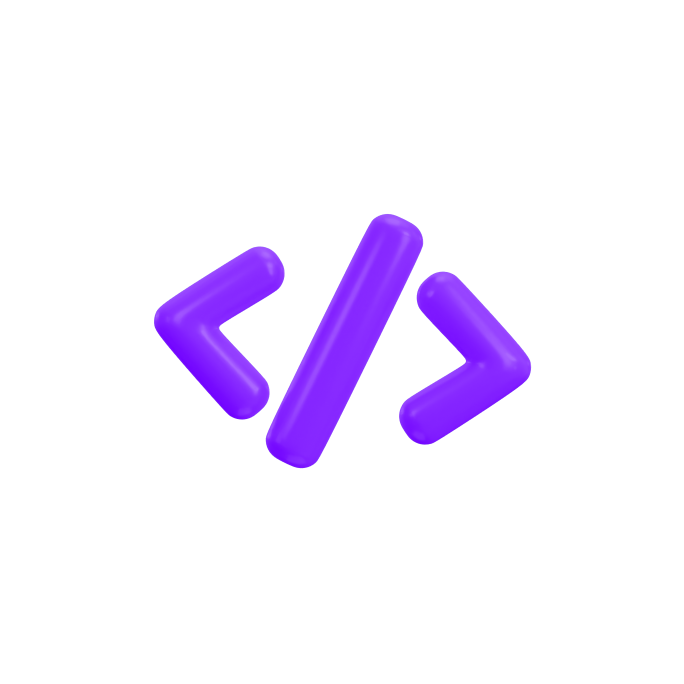
Get developers that understand your business goals
On-time project delivery. Latest coding standards. Data security.
Learn moreConclusion: supercharge your React interfaces
In conclusion, we have found that mastering the art of building efficient user interfaces with React is a transformative skill for any developer.
Knowing from experience, any great, reusable, and interactive UI made with React will have you delving into the power of React components, understanding JSX syntax, and leveraging lifecycle methods.
Just as we took the time to unveil the foundational concepts and techniques necessary to construct exceptional user interfaces, we hope you will take the time to appreciate crafting engaging experiences and pushing the boundaries of what is possible with React.